ESP8266 - Keypad Door Lock
This tutorial instructs you how to build a password door lock system by using ESP8266, keypad, and solenoid lock or electromagnetic lock. The below is how the door lock system will work:
- Users input the password via keypad.
- ESP8266 verifies the password.
- If the inputed password is valid, ESP8266 deactivates the solenoid lock to unlock the door. The door remains unlocked for a specific time (e.g. 20 seconds) before automatically locking again.
- The ESP8266 code supports for multiple passwords.
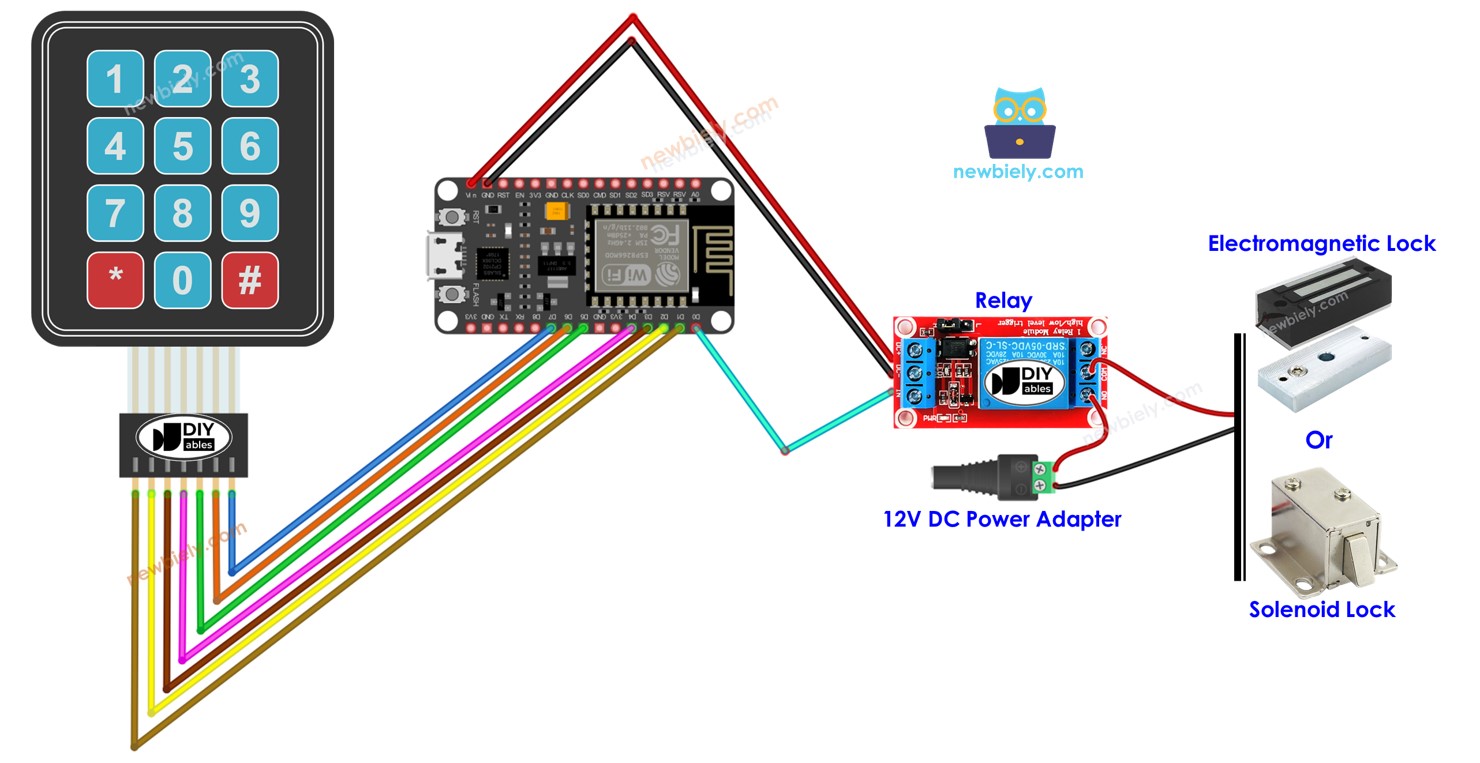
To make it easy, the tutorial is divided into multiple steps, from easy to difficult:
- Part 1: A simple keypad door lock system with Arduino Nano, keypad, solenoid lock or electromagnetic lock.
- Part 2: (Optionally) Adding multiple password supports
- Part 4: (Optionally) Adding the beep sound when the keypad is pressed.
- Part 5: (Optionally) Adding a door sensor to the keypad door lock.
- Part 6: (Optionally) Managing and storing the valid passwords to the internal EEPROM of Arduino Nano
- Part 7: (Optionally) Saving the access history to SD Card
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Keypad, Solenoid Lock, and Electromagnetic Lock
Both the solenoid lock and electromagnetic lock are used to lock/unlock the door. They primarily differ from each other in term of machanical design. Their wiring to ESP8266 are similar. The ESP8266 code for controlling them are the same.
If you are unfamiliar with keypad, solenoid lock, electromagnetic lock (pinout, how it works, how to program ...), the following tutorials can help you learn:
Wiring Diagram
- Wiring Diagram with ESP8266, keypad and solenoid lock
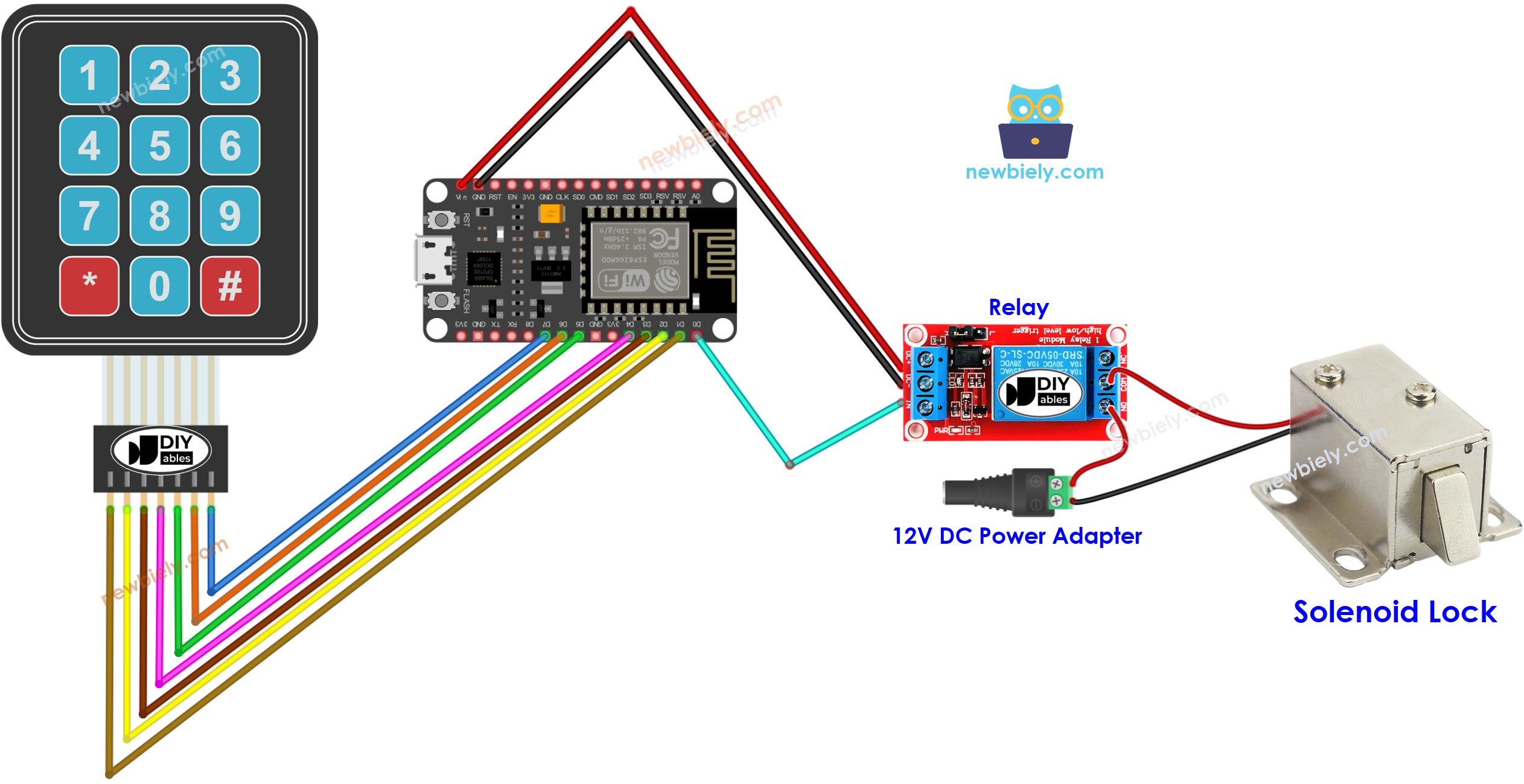
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
- Wiring Diagram with ESP8266, keypad and electromagnetic lock
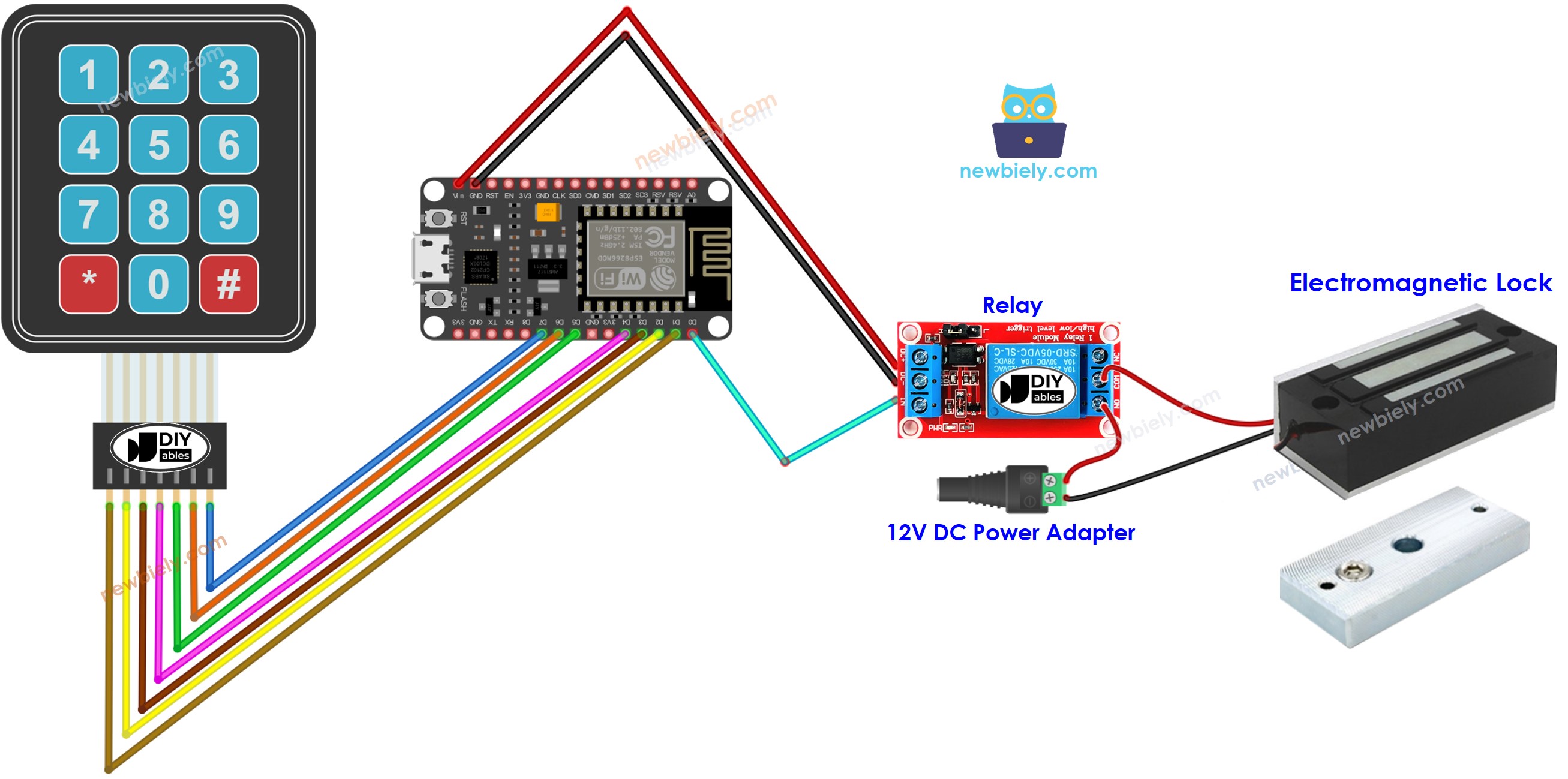
This image is created using Fritzing. Click to enlarge image
ESP8266 Code - Keypad Door Lock
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect a USB cable between the ESP8266 and the PC.
- Open the Arduino IDE, select the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library created by Mark Stanley and Alexander Brevig.
- Then, press the Install button to complete the installation of the keypad library.
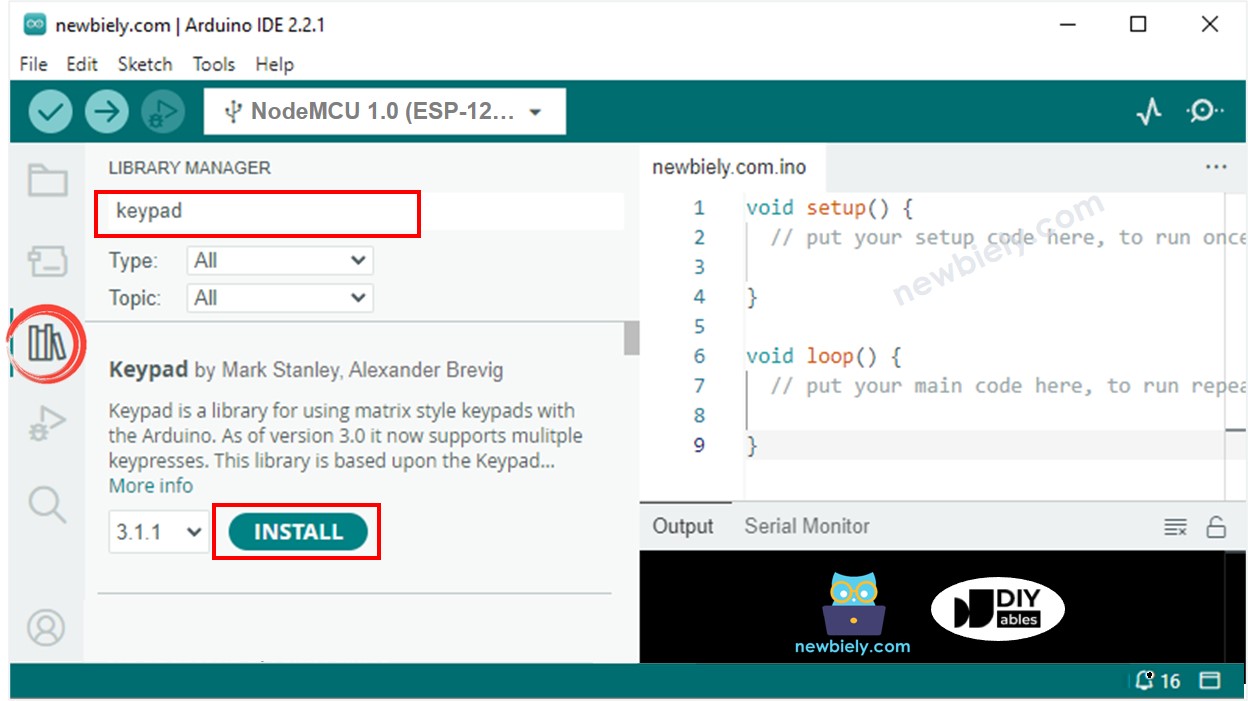
- Copy the code above and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to send the code to the ESP8266.
- Open the Serial Monitor.
- Press the 12345 keys followed by the # symbol.
- Then press the 1234ABC keys followed by the # symbol.
- Check out the lock tongue's state for 20 seconds.
- Check out the result on the Serial Monitor.
Code Explanation
The valid passwords are pre-defined in the ESP8266 code. A string is used to store the input password from users, referred to as input string. In keypad, two keys (* and #) are utilized for special purposes: clear password and terminate password. The system operates as follows:
- Apart from two special keys, if another key is pressed, it is added to the input string
- If * is pressed, input string is cleared. You can use it to initiate or restart inputing the password
- If # is pressed:
- The ESP8266 compares input string with the pre-defined passwords. If it matches with one of the pre-defined passwords, the ESP8266 controls the relay to unlock the door.
- Regardless of whether the password is correct or not, The ESP8266 clears the input string for the next input.
ESP8266 Code - Multiple Keys
Adding the beep sound when the keypad is pressed.
We can make the door lock look responsive by adding a piezo buzzer to generate a short beep each time a key on keypad is pressed.
It is quite simple to add it. So, we're leaving this part up to your creativity. You can check out the ESP8266 - Keypad Beep tutorial for more guidance.
Adding a door sensor to the keypad door lock
In the previously mentioned code, the ESP8266 locks the door after a timeout since unlocking. However, in practical applications, a door sensor is usually added to the system. If the ESP8266 detects that the door is closed, it locks the door immediately instead of waiting for the timeout.
To avoid overwhelming you, we didn't include the door sensor in the wiring diagram and code. Instead, we're leaving this part up to your creativity. You can check out the ESP8266 - Door Sensor and ESP8266 - Door Sensor control Relay tutorials for more guidance.
Managing and storing the valid passwords to EEPROM
The above code has valid passwords (UID) that are hardcoded into the ESP8266 code. This means that if you want to add or remove passwords, you have to make changes to the code and upload it to the ESP8266 again, which is inconvenient.
In real-life applications, it's necessary to manage passwords without having to modify and upload the code every time. To achieve this, the passwords can be stored in EEPROM instead of being hardcoded. Consequently, a method is needed to easily manage the passwords from the EEPROM.
Two methods are available for managing passwords in EEPROM:
- Use master passwords
- By utilizing a password as an ADD master password, new passwords can be added to the system. Once the ESP8266 detects the ADD master password, it switches between the ADD mode and OPERATION mode.
- During the ADD mode, the ESP8266 adds any new passwords it detects to the EEPROM.
- A similar approach is used for DELETE master password and DELETE mode.
- Use a ADD/DELETE commands via Serial/Bluetooth/IR
- Commands are transmitted via Serial/Bluetooth/IR, utilizing tools such as the Serial Monitor, Bluetooth app, or IR controller.
- The commands consist of a directive (ADD/DELETE) and the password.
In order to utilize any of two above methods, a considerable amount of ESP8266 code must be added. For individuals who are new to programming, this can be a challenging task. As a result, this tutorial aims to provide a basic understanding of the door lock system without overwhelming beginners with complex code. If you wish to implement this system for practical purposes, please contact us through our paid programming service
Storing the access history to SD Card
To keep track of access history, it might be necessary to save access status (GRANTED/DENIED), and date and time. Since EEPROM has insufficient capacity to store the entire history, an SD card can be used instead. You can find guidance on this ESP8266 - Log Data with Timestamp to SD Card tutorial.