ESP8266 - Micro SD Card
This tutorial instructs you how to use the Micro SD Card with ESP8266. In detail, we will learn:
- How to connect a Micro SD Card to ESP8266.
- How to program ESP8266 to open a file on the Micro SD Card and create it if it does not already exist.
- How to program ESP8266 to write data to a file on the Micro SD Card.
- How to program ESP8266 to read a file on the Micro SD Card character-by-character.
- How to program ESP8266 to read a file on the Micro SD Card line-by-line.
- How to program ESP8266 to append content to an existing file on the Micro SD Card.
- How to program ESP8266 to overwrite a file on the Micro SD Card.
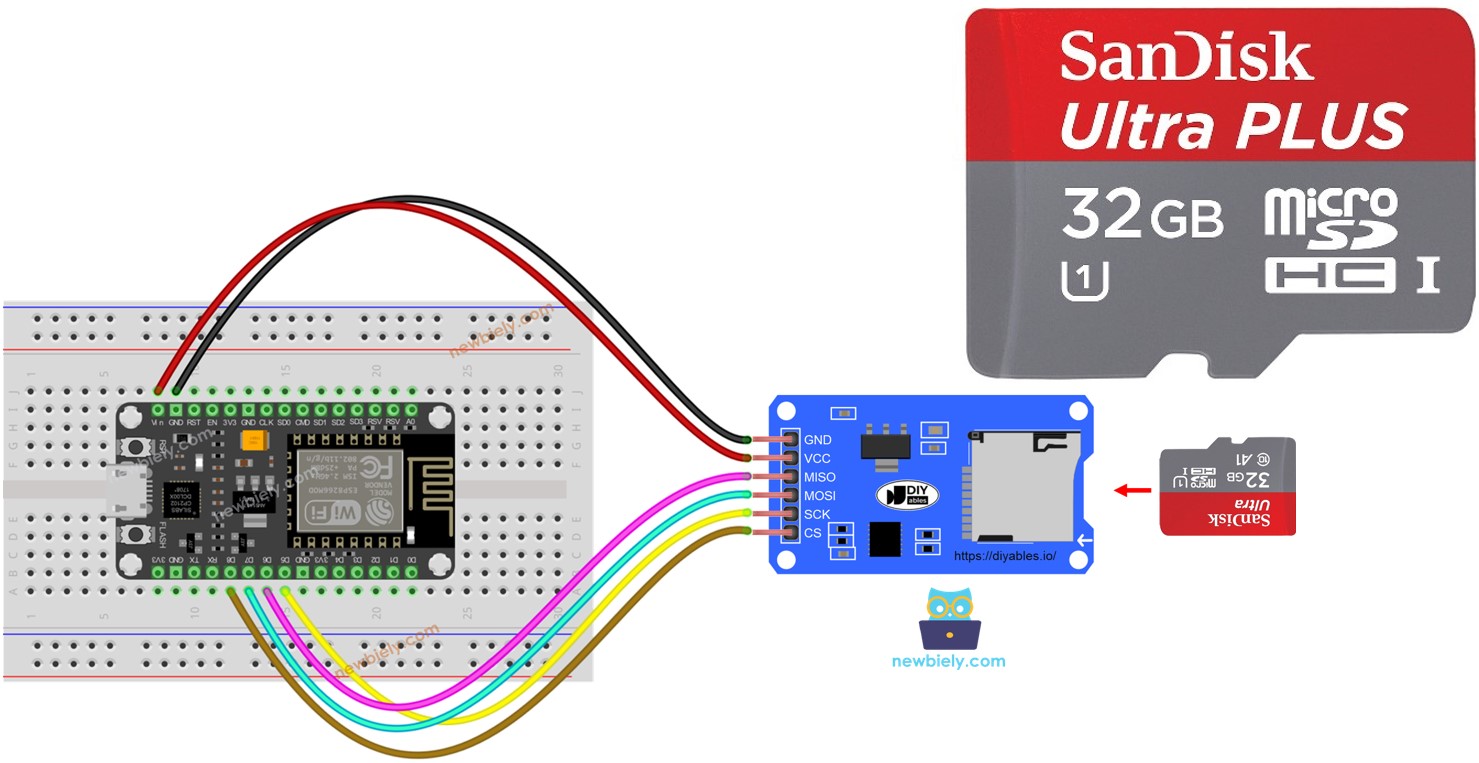
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Micro SD Card Module
The Micro SD Card Module is a link between ESP8266 and a Micro SD Card. It is able to establish a connection with the ESP8266 and can be used to hold the Micro SD Card. In other words, it serves as a bridge between the two.
The Micro SD Card Module Pinout
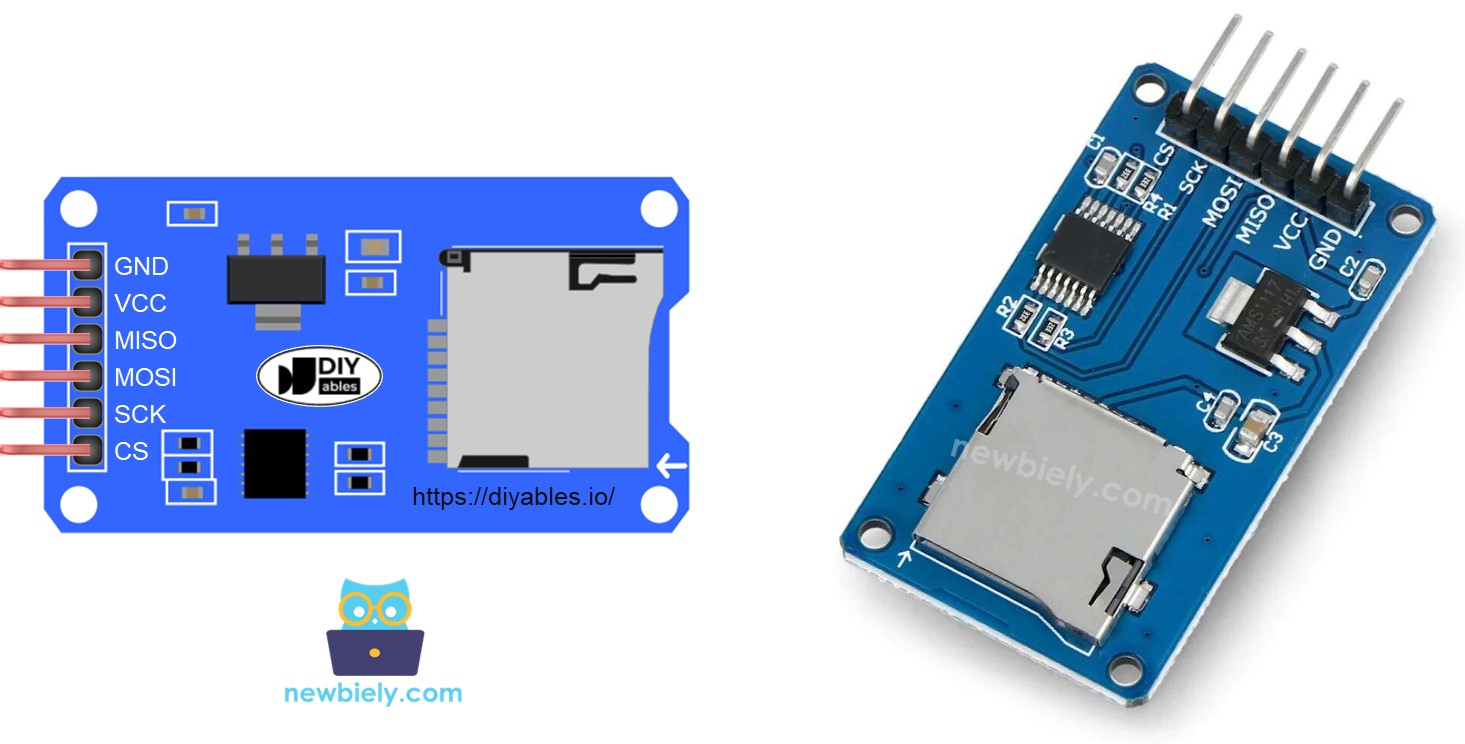
The Micro SD Card Module has 6 pins:
- VCC pin: This should be connected to the Arduino's 5V pin.
- GND pin: This should be connected to the Arduino's GND.
- MISO pin: (Master In Slave Out) This should be connected to the Arduino's MISO pin.
- MOSI pin: (Master Out Slave In) This should be connected to the Arduino's MOSI pin.
- SCK pin: This should be connected to the Arduino's SCK pin.
- SS pin: (Slave Select) This should be connected to the pin specified in ESP8266 code as a SS pin.
Preparation
Ensure that the Micro SD Card is formatted as either FAT16 or FAT32 (you can search online for more information).
Wiring Diagram
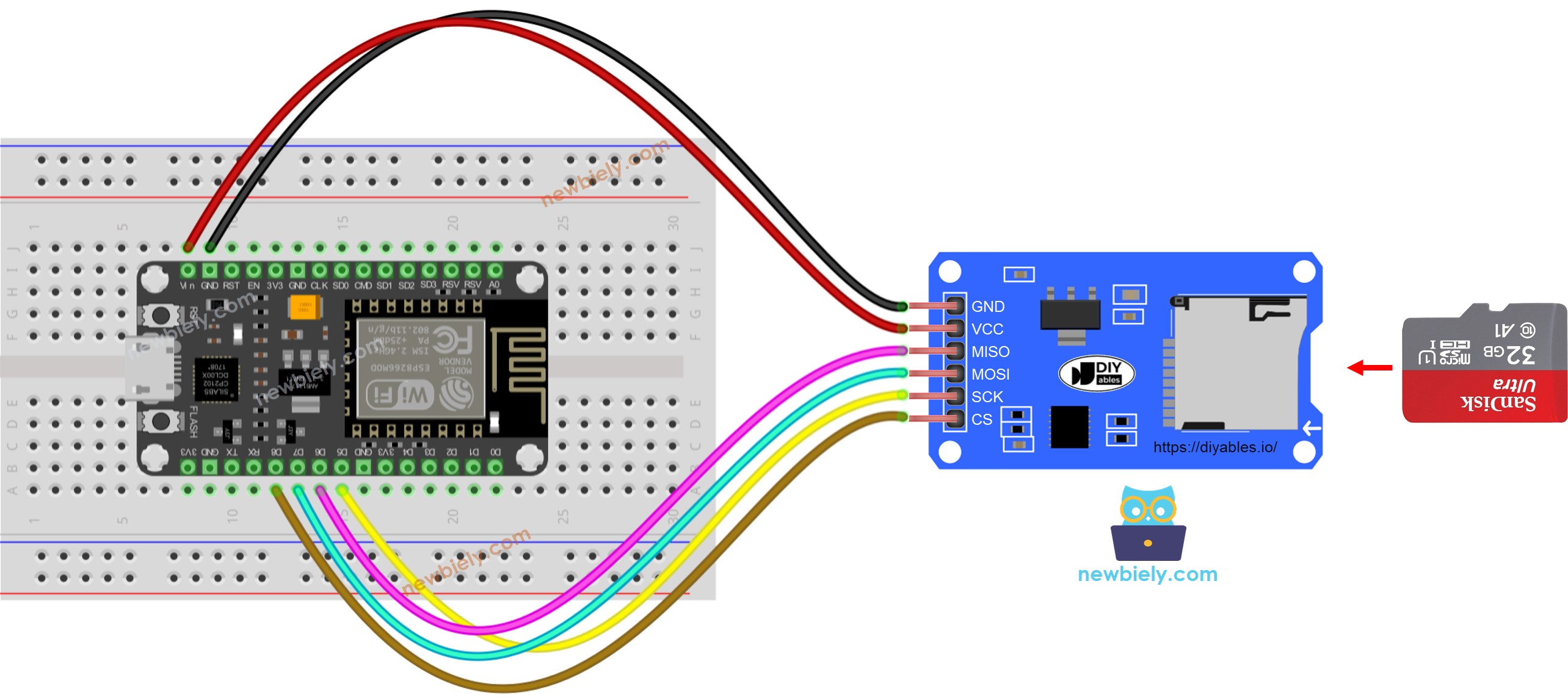
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 - How to open a file on Micro SD Card and create if not existed
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Insert the Micro SD Card into the Micro SD Card module.
- Make the connections between the Micro SD Card module and ESP8266 as per the wiring diagram.
- Connect the ESP8266 to the PC with a USB cable.
- Open the Arduino IDE and select the appropriate board and port.
- Open the Serial Monitor in the Arduino IDE.
- Copy and paste the following code into the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
- The Serial Monitor will show the result of the first execution.
- Will be different
- The outcome displayed on the Serial Monitor for subsequent executions will be distinct.
※ NOTE THAT:
You may not observe the output on the Serial Monitor when you first upload your code, if it is done before opening the Serial Monitor.
- Remove the Micro SD Card from the module.
- Put the Micro SD Card into a USB SD Card reader.
- Attach the USB SD Card reader to the computer.
- Verify if the file is present or not.
ESP8266 - How to write/read data to/from a file on Micro SD Card
The following code:
- Saves data to a file
- Reads the content of the file character-by-character and displays it on the Serial Monitor
- The Serial Monitor displayed the contents of the file.
※ NOTE THAT:
By default, the data will be added to the end of the file. If you restart ESP8266 with the code above, the text will be appended to the file again, and the Serial Monitor will display more lines like this:
You can remove the Micro SD Card from the module and use a USB SD Card reader to view its contents on your computer.
ESP8266 - How to read a file on Micro SD Card line-by-line
- The output displayed on the Serial Monitor.
※ NOTE THAT:
You may observe additional lines on Serial Monitor if the file's content has not been erased previously.
ESP8266 - How to overwrite a file on Micro SD Card
By default, the content will be added to the end of the file. The most straightforward way to overwrite a file is: delete the existing file and create a new one with the same name.
- The outcome displayed on the Serial Monitor.
- Reboot the ESP8266
- Verify if the material in the file is added to the Serial Monitor or not.
You can remove the Micro SD Card from the module and view its contents on your computer. A USB SD Card reader is necessary for this.