ESP8266 - Motion Sensor - Servo Motor
This tutorial instructs you how to use ESP8266 and motion sensor to control servo motor. In detail:
- When motion is detected, ESP8266 rotates servo motor to 90 degree
- When motion is not detected, ESP8266 rotates servo motor back to 0 degree.
This can be applied in an automation process that triggers actions upon detecting human presence.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Servo Motor and Motion Sensor
If you are unfamiliar with servo motor and motion sensor (including pinout, functioning, programming, etc.), the following tutorials can help:
Wiring Diagram
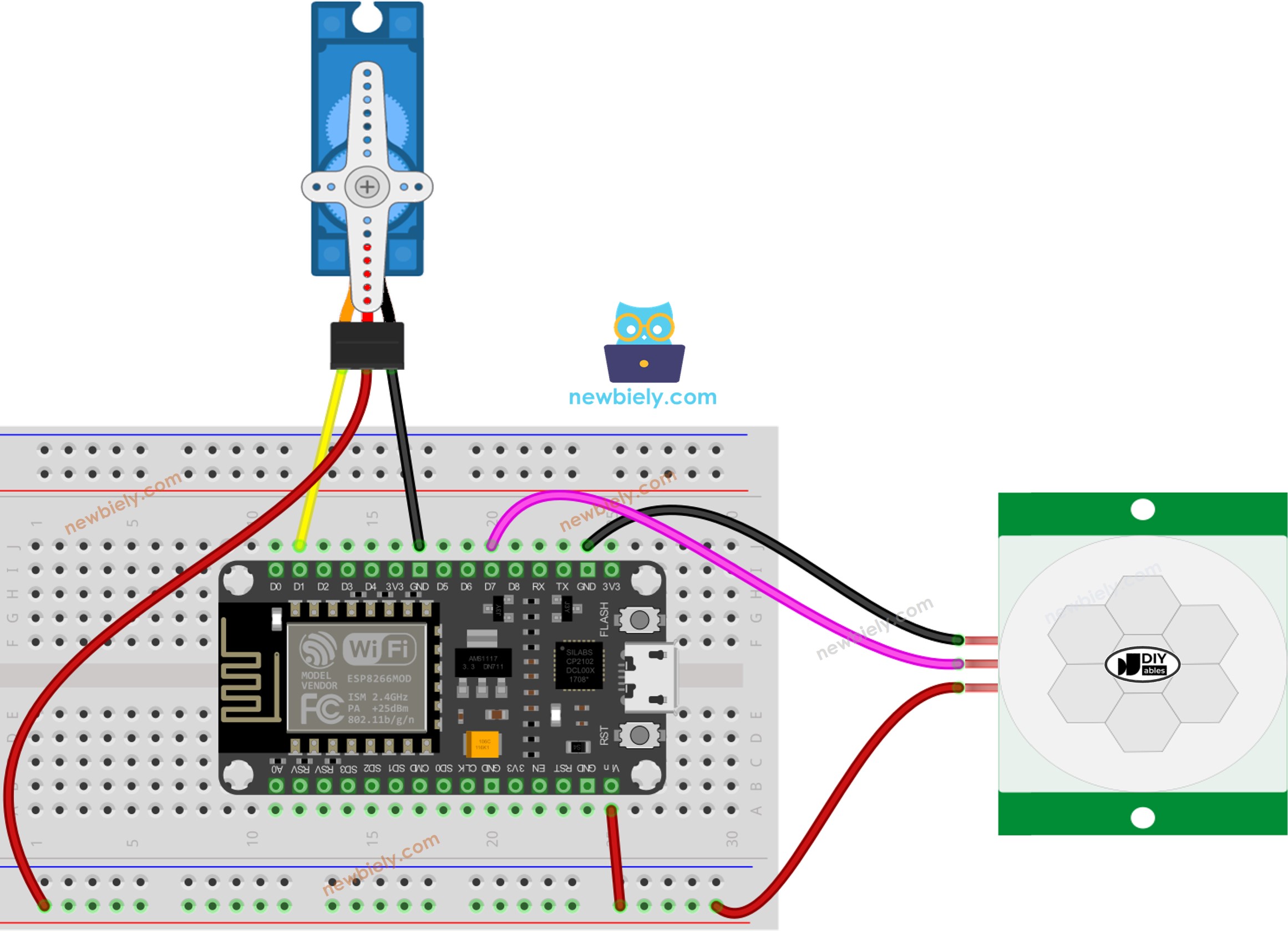
This image is created using Fritzing. Click to enlarge image
Please note that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to provide more power for the servo motor. The below demonstrates the wiring diagram with an external power source for servo motor.
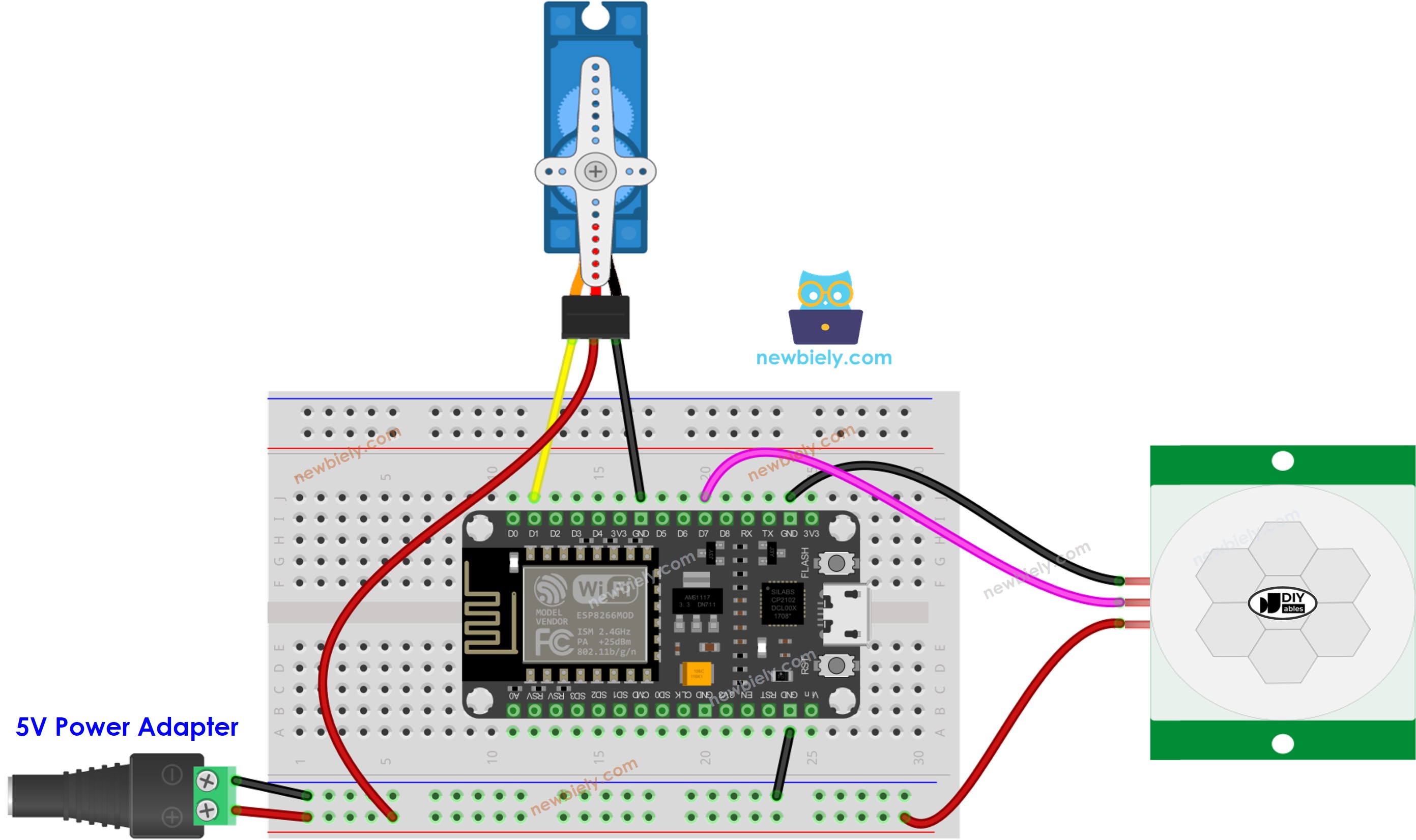
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Please do not forget to connect GND of the external power to GND of ESP8266.
Initial Setting
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
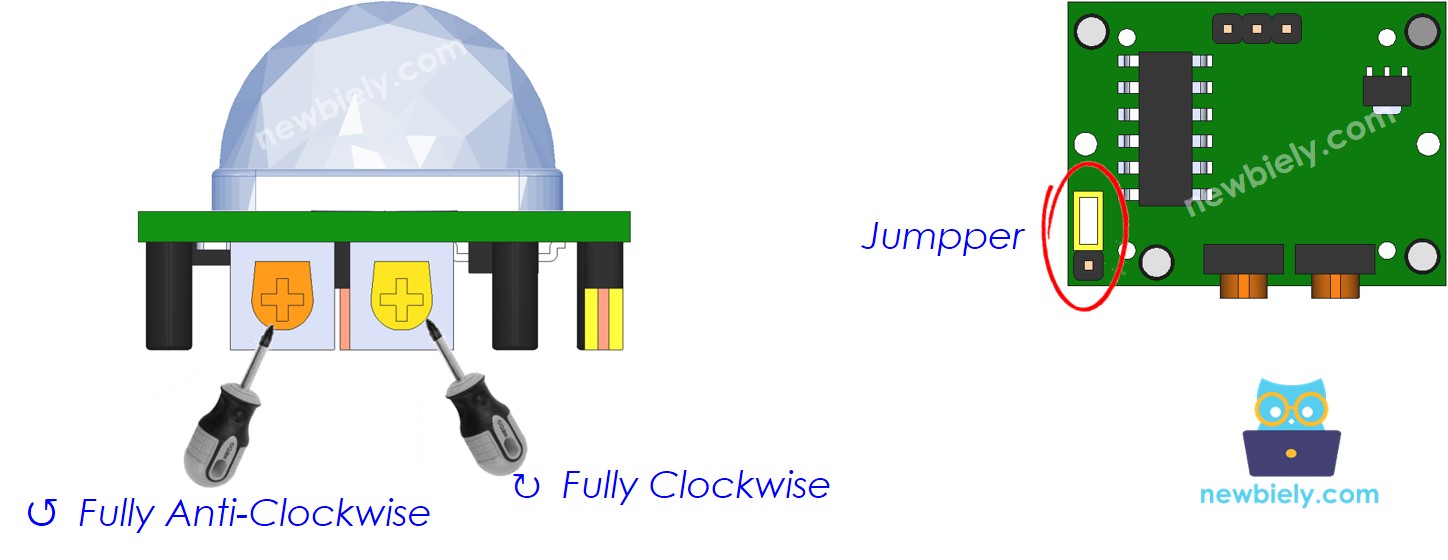
ESP8266 Code - Motion Sensor Controls Servo Motor
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect your ESP8266 to a computer using a USB cable.
- Launch the Arduino IDE, select the appropriate board and port.
- Paste the code into the IDE and open it.
- Click the Upload button on the IDE to send the code to the ESP8266.
- Move your hand in front of the sensor.
- Check out the servo motor's movements.