ESP8266 - Keypad - LCD
This tutorial instructs you how to use ESP8266 to display the input from keypad on LCD display.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad and LCD
If you are unfamiliar with keypad and LCD (pinout, how it works, how to program ...), the following tutorials can help you understand them:
Wiring Diagram
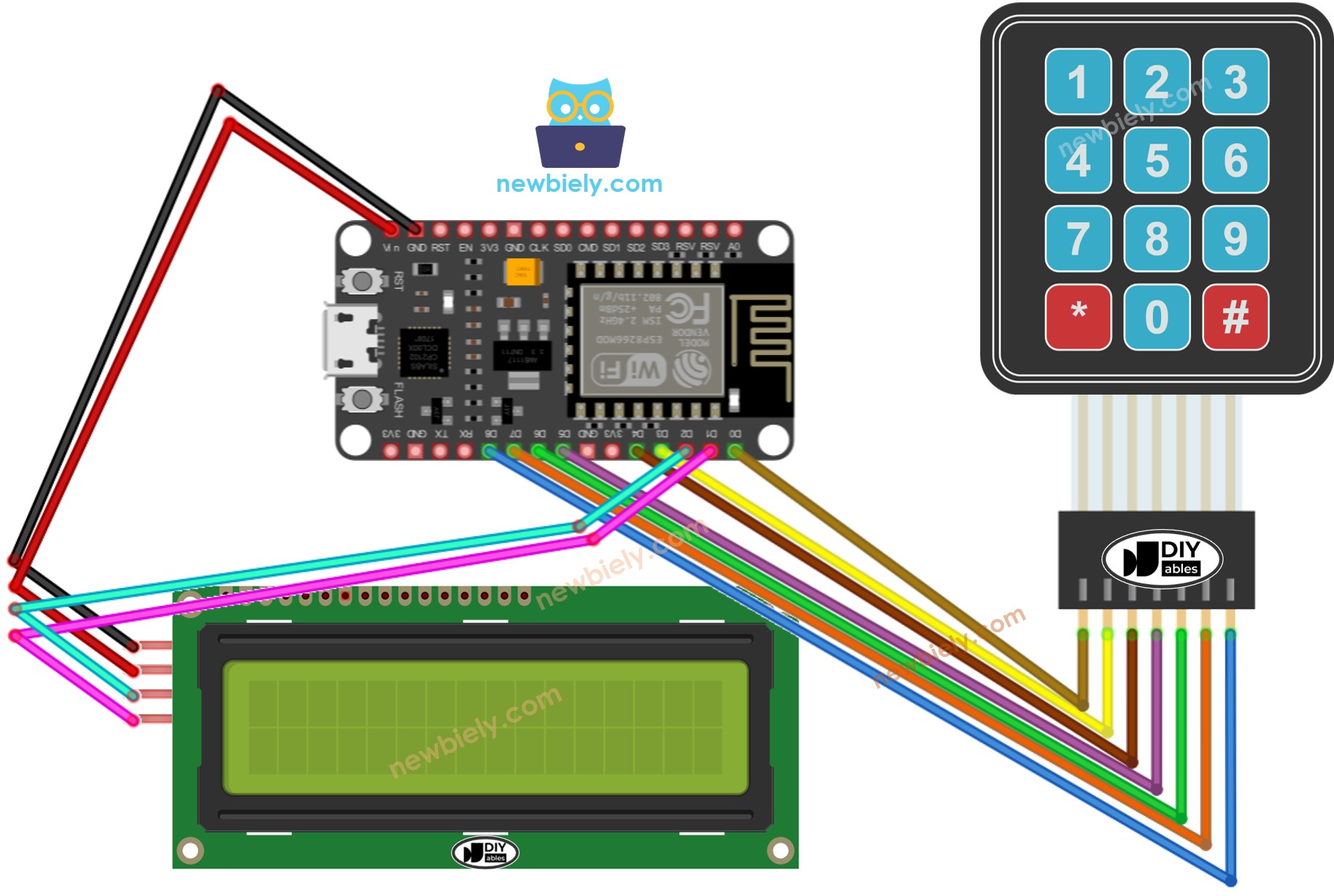
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code
※ NOTE THAT:
The address of the LCD may differ depending on the manufacturer. In our code, we have used 0x27 which is specified by DIYables manufacturer.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect an USB cable between the ESP8266 and the PC.
- Open the Arduino IDE, select the appropriate board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library created by Mark Stanley and Alexander Brevig.
- Then, press the Install button to complete the installation of the keypad library.
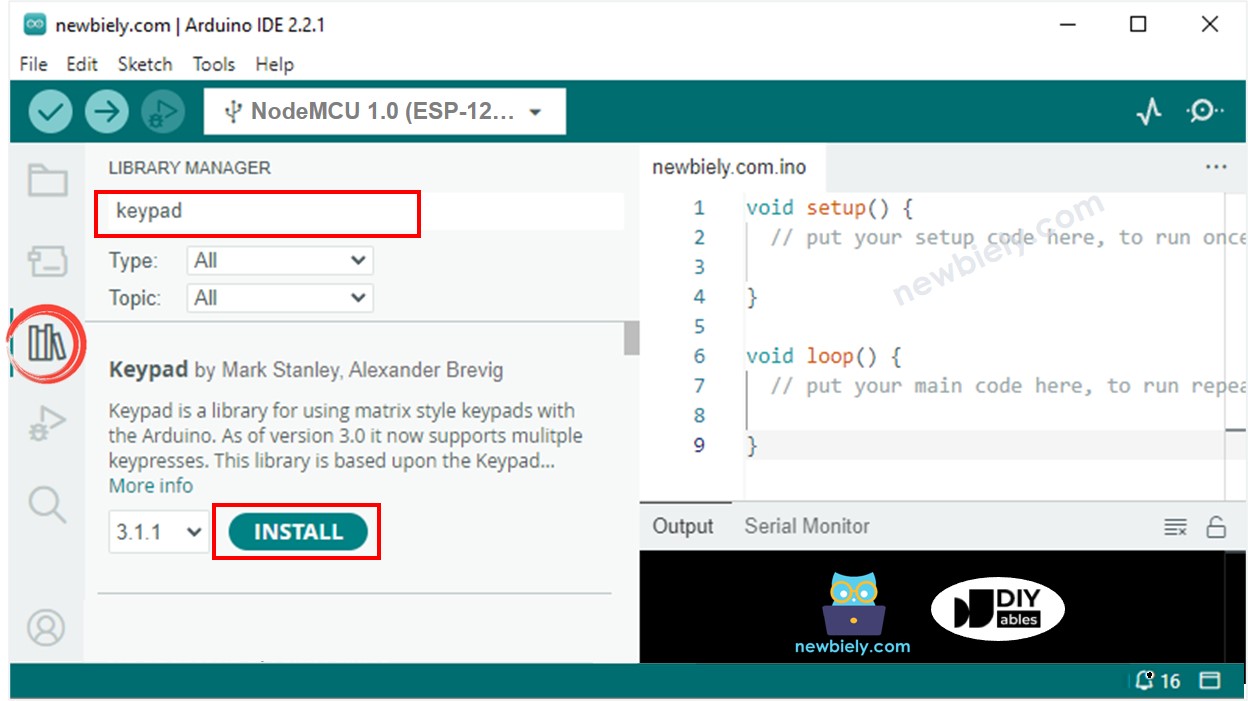
- Search for “LiquidCrystal I2C” and locate the LiquidCrystal_I2C library by Frank de Brabander.
- Then, click the Install button to install it.
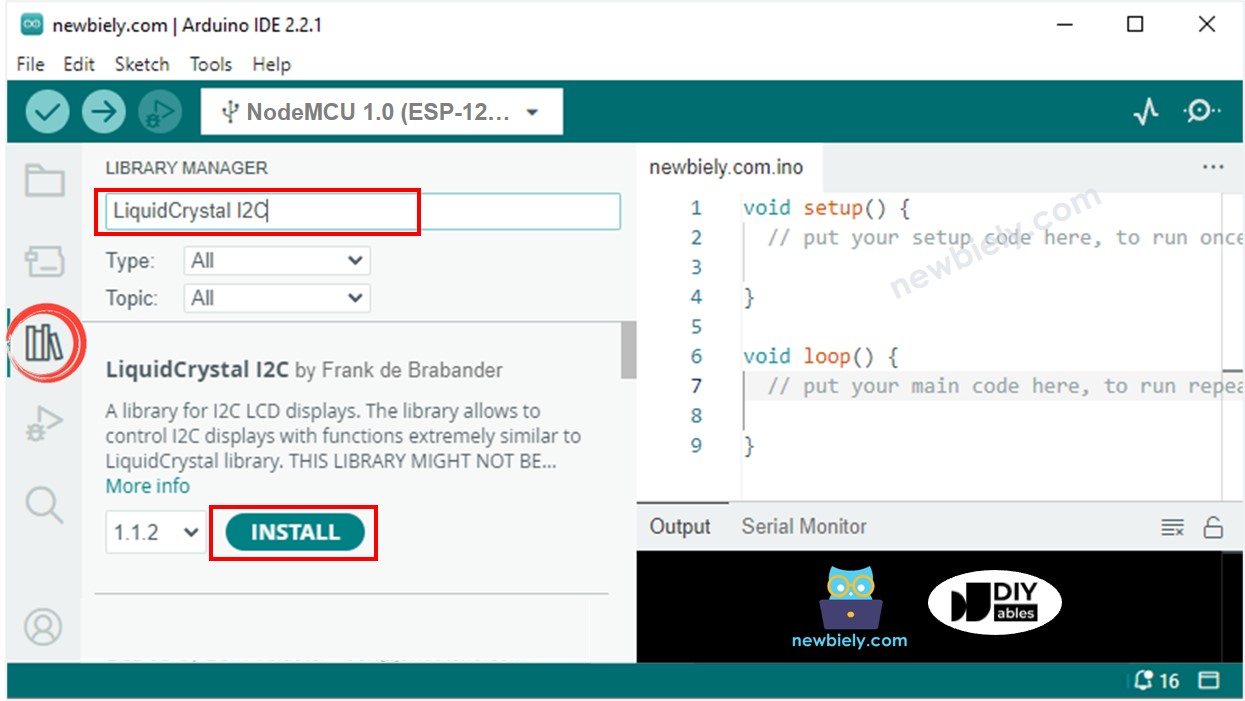
Because the ESP8266 pins are not sufficient, We need to use the pin D8 for the keypad. As mentioned in the ESP8266 - Keypad tutorial, when using the ESP8266 pin D8 for a column pin of keypad, we MUST modify the Keypad library as follows:
- Find the Arduino\libraries\Keypad\src\Keypad.cpp file, go to line 98, where it looks like this: pin_mode(columnPins[c], INPUT);
- Comment out this line. After modification, the code at line 98 should look like this: //pin_mode(columnPins[c], INPUT);
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and upload the code to the ESP8266.
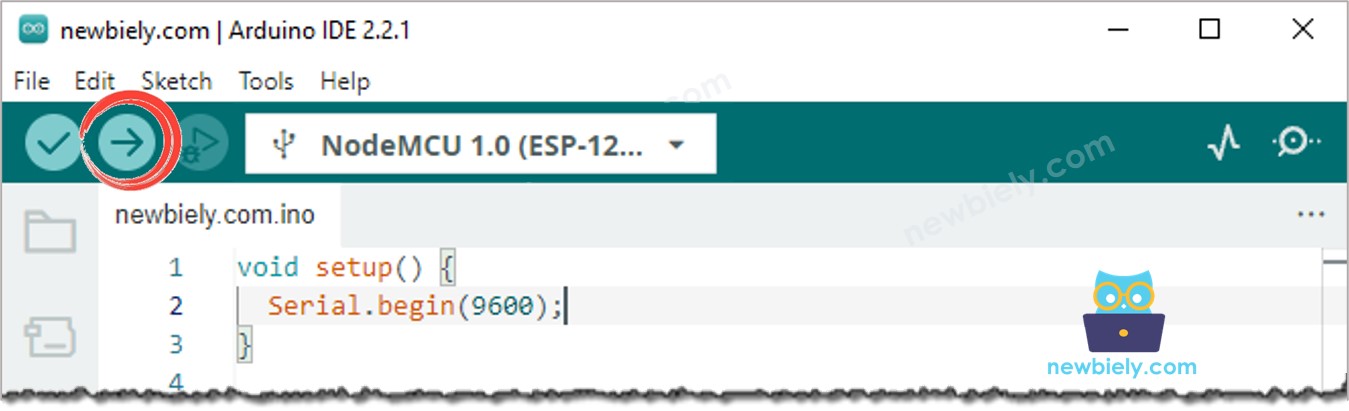
- Press certain keys on the keypad and
- Observe the result displayed on the LCD.
If the LCD display is not showing anything, please refer to Troubleshooting on LCD I2C . for assistance.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!