ESP8266 - Web Server Password
This tutorial instructs you on how to make a safe ESP8266 web server that asks a username and password for access. Prior to viewing any web pages stored on the ESP8266, users will need to input their username and password.
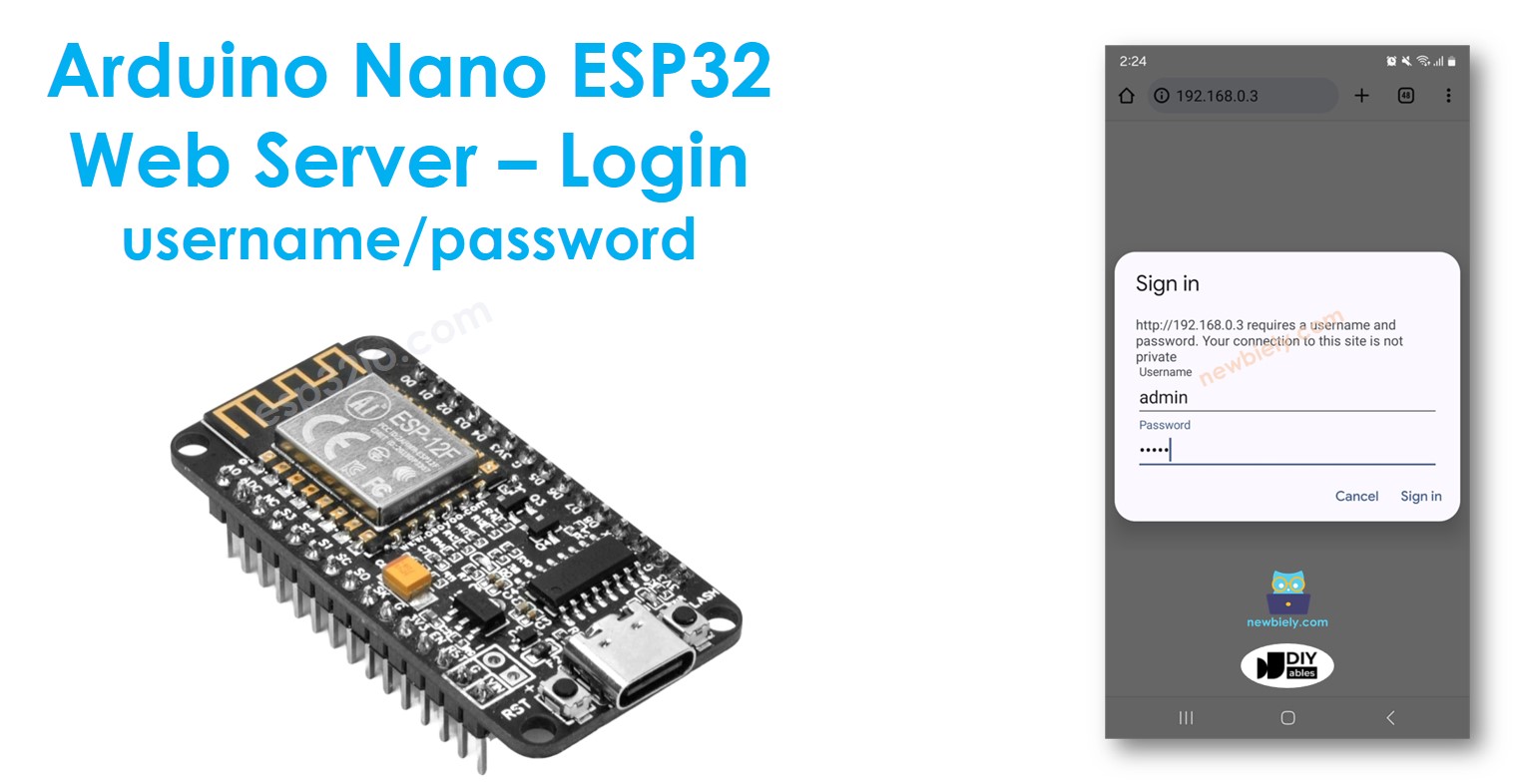
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of ESP8266 and Web Server
If you're not familiar with ESP8266 and Web Server (including pinout, how it works, and programming), you can learn about them through the following tutorials:
ESP8266 Code - Web server Username/Password
/*
* This ESP8266 NodeMCU code was developed by newbiely.com
*
* This ESP8266 NodeMCU code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/esp8266/esp8266-web-server-password
*/
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
// Replace with your network credentials
const char* ssid = "YOUR_WIFI_SSID"; // CHANGE IT
const char* password = "YOUR_WIFI_PASSWORD"; // CHANGE IT
ESP8266WebServer server(80);
const char* www_username = "admin";
const char* www_password = "esp8266";
void handleRoot() {
if (!server.authenticate(www_username, www_password)) {
return server.requestAuthentication();
}
// send your web content here. The below is simplest form.
server.send(200, "text/html", "<html><body><h1>Login Successful!</h1><p>You are now logged in.</p></body></html>");
}
void handleNotFound() {
server.send(404, "text/plain", "Not found");
}
void setup() {
Serial.begin(9600);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
server.on("/", HTTP_GET, handleRoot);
server.onNotFound(handleNotFound);
server.begin();
// Print the ESP8266's IP address
Serial.print("ESP8266 Web Server's IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
server.handleClient();
}
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to ESP8266
- Open the Serial Monitor
- Check out the result on Serial Monitor.
COM6
Connecting to WiFi...
Connected to WiFi
ESP8266 Web Server's IP address: 192.168.0.3
Autoscroll
Clear output
9600 baud
Newline
- You will see an IP address on the Serial Monitor, for example: 192.168.0.3
- Type the IP address on the address bar of a web browser on your smartphone or PC.
- Please note that you need to change the 192.168.0.3 to the IP address you got on Serial Monitor.
- You will see a page that promont you to input username/password.
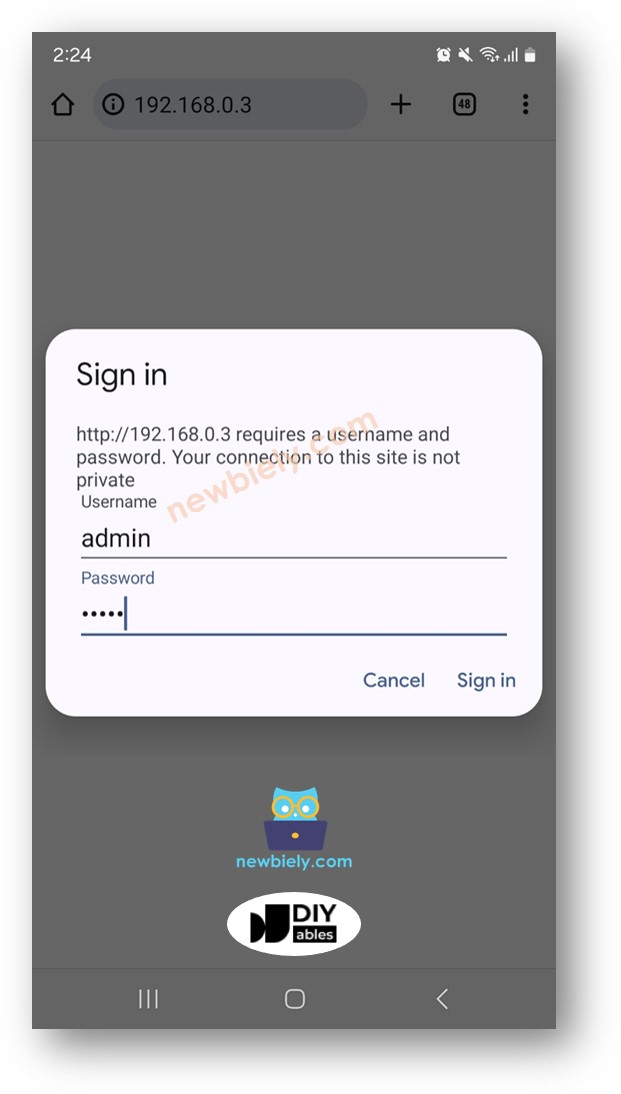
- Type username/password that are in the ESP8266 code, in this case: admin as username, esp8266 as password
- If you input the username/password correctly, the web content of ESP8266 will be shown:
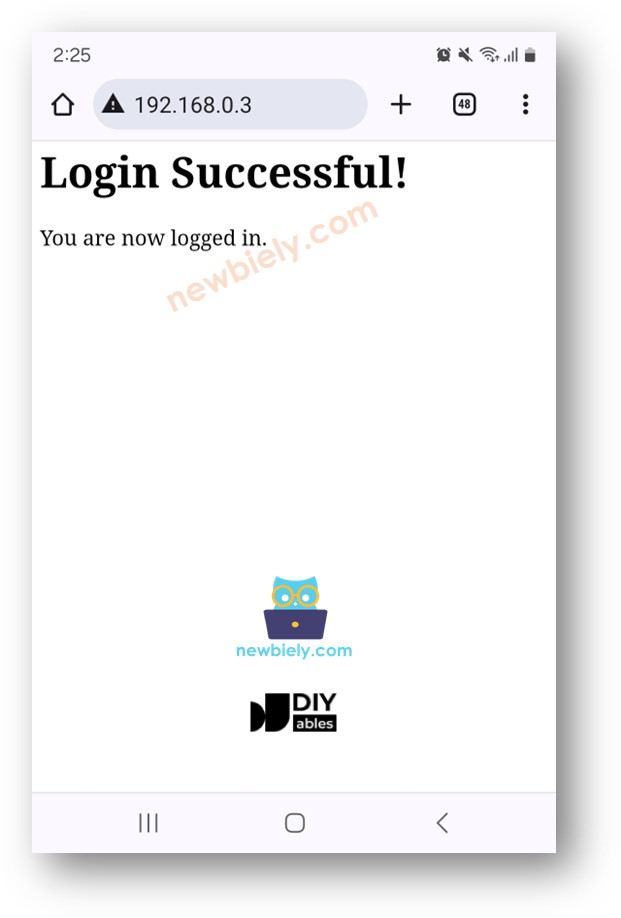
※ NOTE THAT:
- You can modify the username and password for your website directly within the code by changing the values assigned to two variables: www_username and www_password.
- You have the freedom to personalize this code by integrating your own HTML, CSS, and JavaScript to craft your webpage to your liking.
- It's worth mentioning that there's no specific HTML code within the code itself for the login form where users input their username and password. This might seem unexpected, but instead, the login form is created dynamically by the web browser when necessary.
Advanced Knowledge
This segment offers a detailed explanation of how the username/password system functions without HTML for the login form:
- When you enter the IP address of the ESP8266 into a web browser for the first time, the browser sends a request to the ESP8266 via HTTP, but without any username/password information.
- Upon receiving this request, the ESP8266's code checks if there are any username/password credentials provided. If none are detected, the ESP8266 does not reply with the content of the page. Instead, it sends back an HTTP message containing headers instructing the browser to request the user's username/password. Notably, this response does not include HTML code for the login form.
- When the browser receives this response, it interprets the HTTP headers and understands that the ESP8266 is asking for a username/password. Consequently, the browser dynamically generates a login form for the user to enter their credentials.
- The user then inputs their username/password into this form.
- The browser incorporates the entered username/password into an HTTP request and forwards it back to the ESP8266.
- The ESP8266 verifies the username/password provided in the HTTP request. If they are correct, it supplies the content of the requested page. If they are incorrect, the process repeats, prompting the user to enter the correct credentials once more.
- Once the user correctly inputs their username/password for the first time, subsequent requests do not require them to re-enter the details. This is because the browser automatically stores the credentials and includes them in subsequent requests.