ESP8266 - DRV8825 Stepper Motor Driver
In this guide, we will learn about the DRV8825 Stepper Motor Driver and how to use it with ESP8266 to operate the stepper motor. Here are the details we will cover:
- What the DRV8825 Stepper Motor Driver Module Is
- How the DRV8825 Stepper Motor Driver Module Functions
- Connecting the DRV8825 Stepper Motor Driver to ESP8266 and a Stepper Motor
- Programming ESP8266 to Manage a Stepper Motor with the DRV8825 Module
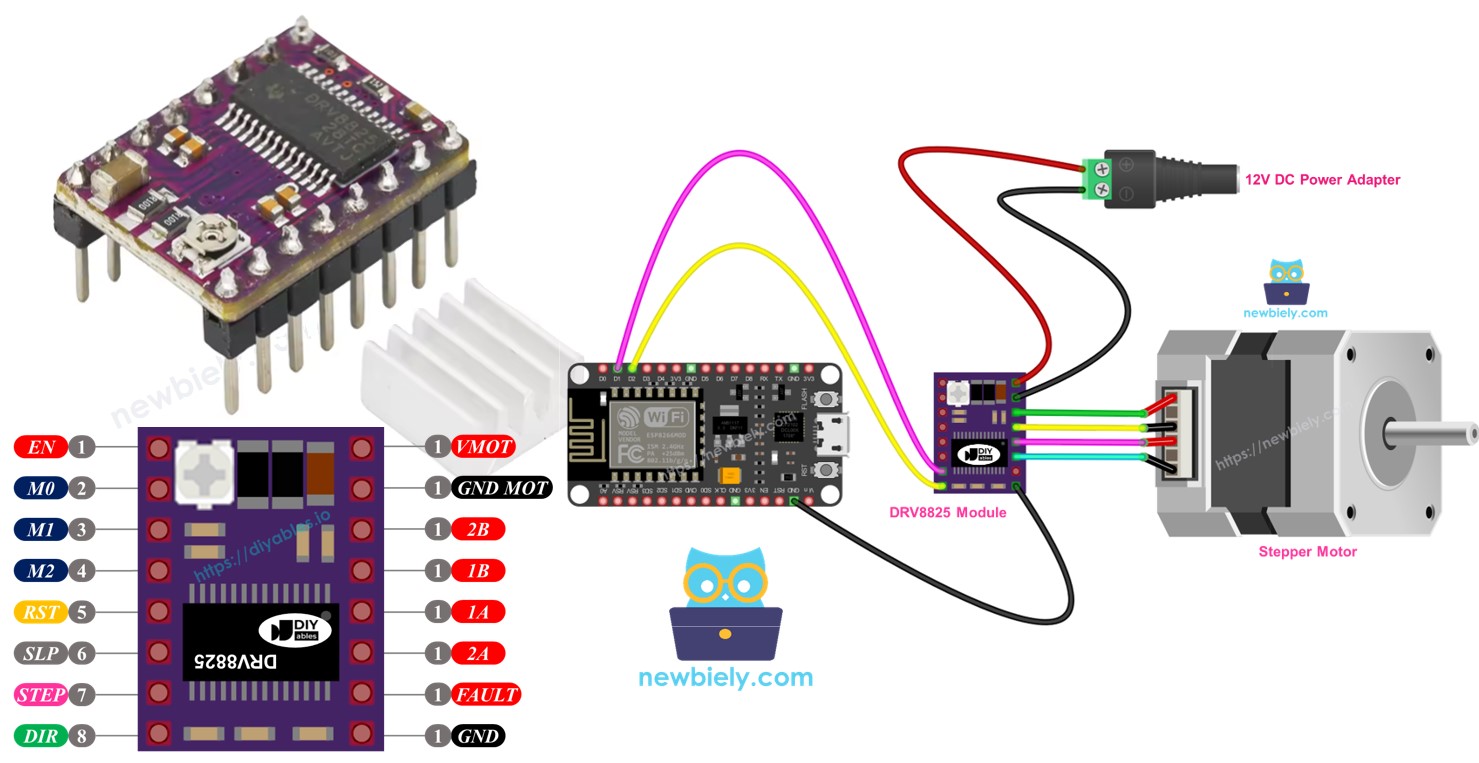
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DRV8825 Stepper Motor Driver
The DRV8825 is a popular module for managing bipolar stepper motors, often used in CNC machines, 3D printers, and robots. It has an adjustable current control, protection against overheating, and provides several microstepping choices like full-step, half-step, and fractions down to 1/32 step. This module can manage up to 2.2A for each coil with adequate cooling and works within a broad voltage range from 8.2V to 45V, accommodating different stepper motors.
To understand stepper motor concepts such as full-step, microstepping, unipolar stepper, and bipolar stepper, check out the ESP8266 - Stepper Motor guide.
It's amazing that you only need two ESP8266 pins to manage how fast and in what direction a bipolar stepper motor, like the NEMA 17, moves.
DRV8825 Stepper Motor Driver Pinout
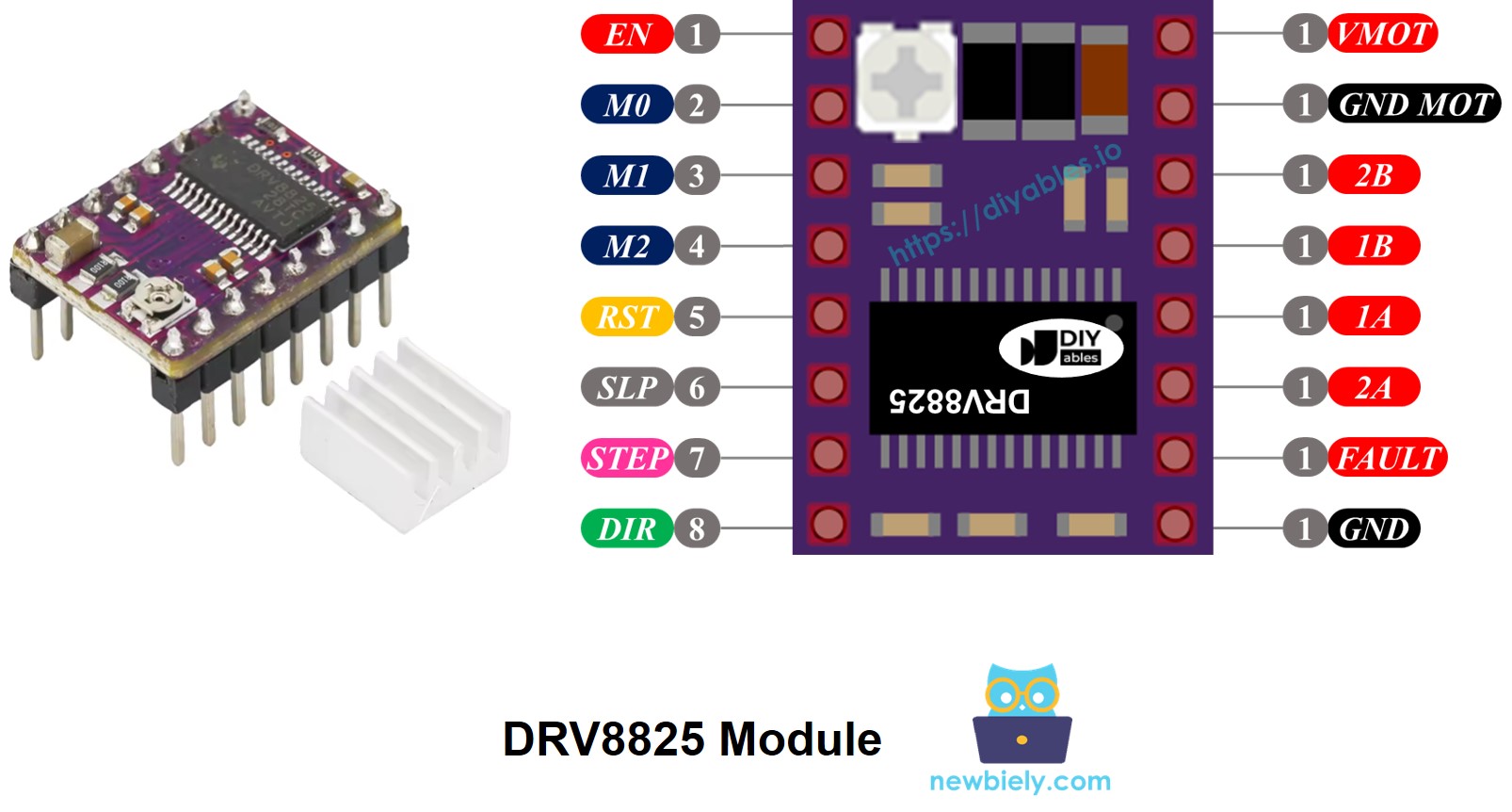
The DRV8825 Stepper motor driver features 16 pins. Here is a common arrangement for the pins on the DRV8825 stepper motor driver module. Keep in mind that some versions of the module might name the pins a bit differently, but their roles are unchanged.
Pin Name | Description |
---|---|
VMOT | Motor power supply (8.2 V to 45 V). This powers the stepper motor. |
GND (for Motor) | Ground reference for the motor power supply. Connect this pin to the GND of the motor power supply |
2B, 2A | Outputs to Coil B of the stepper motor. |
1A, 1B | Outputs to Coil A of the stepper motor. |
FAULT | Fault Detection Pin. This is an output pin that drives LOW whenever the H-bridge FETs are disabled as the result of over-current protection or thermal shutdown. |
GND (for Logic) | Ground reference for the logic signals. Connect this pin to the GND of ESP8266 |
ENABLE | Active-Low pin to enable/disable the motor outputs. LOW = Enabled, HIGH = Disabled. |
M1, M2, M3 | Microstepping resolution selector pins (see table below). |
RESET | Active-Low reset pin - pulling this pin LOW resets the driver. |
SLEEP | Active-Low sleep pin - pulling this pin LOW puts the driver into low-power sleep mode. |
STEP | Step input - a rising edge on this pin advances the motor by one step (or one microstep, depending on microstepping setting). |
DIR | Direction input - sets the rotation direction of the stepper motor. |
Also, there is a tiny built-in dial that you can turn to adjust the current control, which helps stop the stepper motor and driver from getting too hot.
In short, these 16 pins are grouped into these types based on their use:
- Wires connected to the stepper motor: 1A, 1B, 2A, 2B.
- Wires connected to ESP8266 for controlling the driver: ENABLE, M1, M2, M3, RESET, SLEEP.
- Wires connected to ESP8266 for controlling motor direction and speed: DIR, STEP.
- Wire for sending feedback to ESP8266: FAULT.
- Wires connected to the motor's power source: VMOT, GND (motor power ground).
- Wire connected to the ESP8266's ground: GND (logic ground).
The DRV8825 unit doesn't need a separate power source from the ESP8266, because it gets its power directly from the motor's power supply with its built-in 3.3V voltage regulator. However, you must connect the ESP8266's ground to the DRV8825's GND (logic) pin to make sure it works correctly and the ground level is the same for both devices.
Microstep Configuration
The DRV8825 driver allows microstepping by breaking each step into smaller parts. It does this by sending different amounts of current to the motor coils.
For instance, NEMA 17 motor with a 1.8° step angle (200 steps per turn):
- Full-step mode: 200 steps per revolution
- Half-step mode: 400 steps per revolution
- Quarter-step mode: 800 steps per revolution
- Eighth-step mode: 1600 steps per revolution
- Sixteenth-step mode: 3200 steps per revolution
- Thirty-second-step mode: 6400 steps per revolution
When you raise the microstepping level, the motor operates more smoothly and accurately, but it needs more steps per revolution. If you keep the same step pulse rate, then each revolution will last longer, causing the motor to slow down.
If your microcontroller can send pulses fast enough to match the higher step count, you can keep or even boost speed. The real limit relies on how quickly both the driver and your microcontroller can handle these pulses without missing steps.
DRV8825 Microstep Selection Pins
The DRV8825 has three inputs for choosing microstep resolution called M0, M1, and M2 pins. You can set these pins at certain logic levels to select from six different microstepping resolutions:
M0 Pin | M1 Pi | M2 Pi | Microstep Resolution |
---|---|---|---|
Low | Low | Low | Full step |
High | Low | Low | Half step |
Low | High | Low | 1/4 step |
High | High | Low | 1/8 step |
Low | Low | High | 1/16 step |
High | Low | High | 1/32 step |
Low | High | High | 1/32 step |
High | High | High | 1/32 step |
These small setting pins have built-in resistors that pull them down to a LOW state normally. If not connected, the motor will run in full-step mode.
How it Works
To operate a stepper motor with the DRV8825 module, you require at least two ESP8266 pins: one for the DIR pin and another for the STEP pin. The DRV8825 processes these signals from the ESP8266 to accurately move the stepper motor.
- STEP Pin: Each signal on the STEP pin moves the motor one tiny step or a full step, based on your settings.
- DIR Pin: Sets which way the motor spins.
The driver uses these signals and its settings to send control signals to the motor via the 1A, 1B, 2A, and 2B pins.
You can also set up extra pins on the DRV8825 module (ENABLE, M1, M2, M3, RESET, SLEEP) in one of three methods:
- Let them stay separate for the driver to use basic settings.
- Attach them directly to GND or VCC to set a constant mode.
- Link them to ESP8266 pins to manage these functions through your code.
Wiring Diagram between ESP8266, DRV8825 module and Stepper Motor
The diagram below displays the basic connections required between the ESP8266, DRV8825 module, and the stepper motor. With this arrangement, the DRV8825 driver functions in its standard mode (full-step).
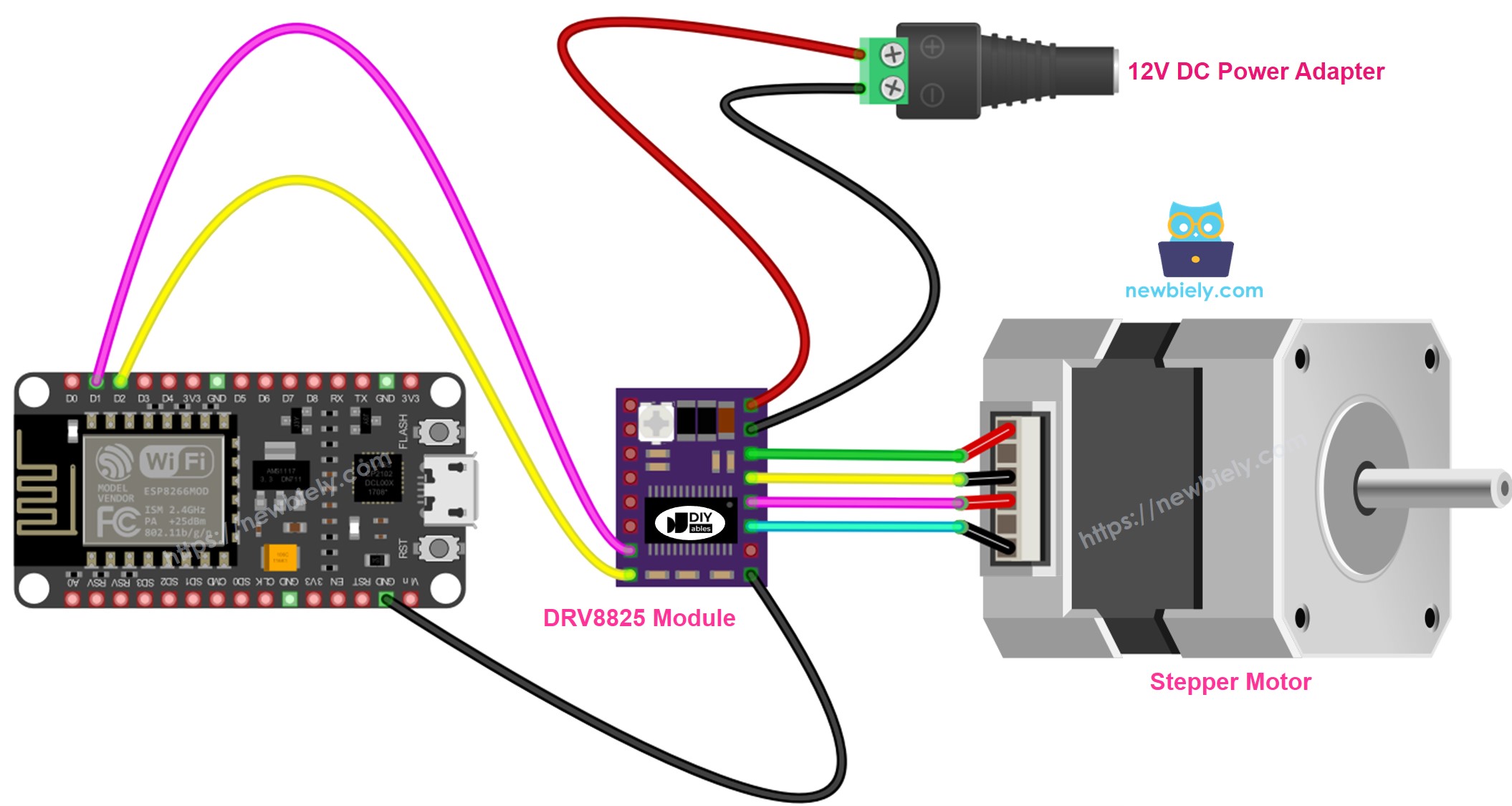
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
In a detailed way:
- VMOT: Connect to the motor's power source, such as 12V.
- GND (for Motor): Attach to the motor power supply's ground.
- 1A, 1B, 2A, 2B: Attach to the coils of the stepper motor.
- STEP: Connect to digital pin D4 on the ESP8266.
- DIR: Connect to digital pin D3 on the ESP8266.
- GND (for Logic): Connect to the GND pin on the ESP8266.
- Other pins: Do not connect.
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code above and open it in the ESP8266 software.
- Click on the Libraries icon on the left side of the ESP8266 interface.
- Type "AccelStepper" in the search box, and look for the AccelStepper library by Mike McCauley.
- Press the Install button to add the AccelStepper library.
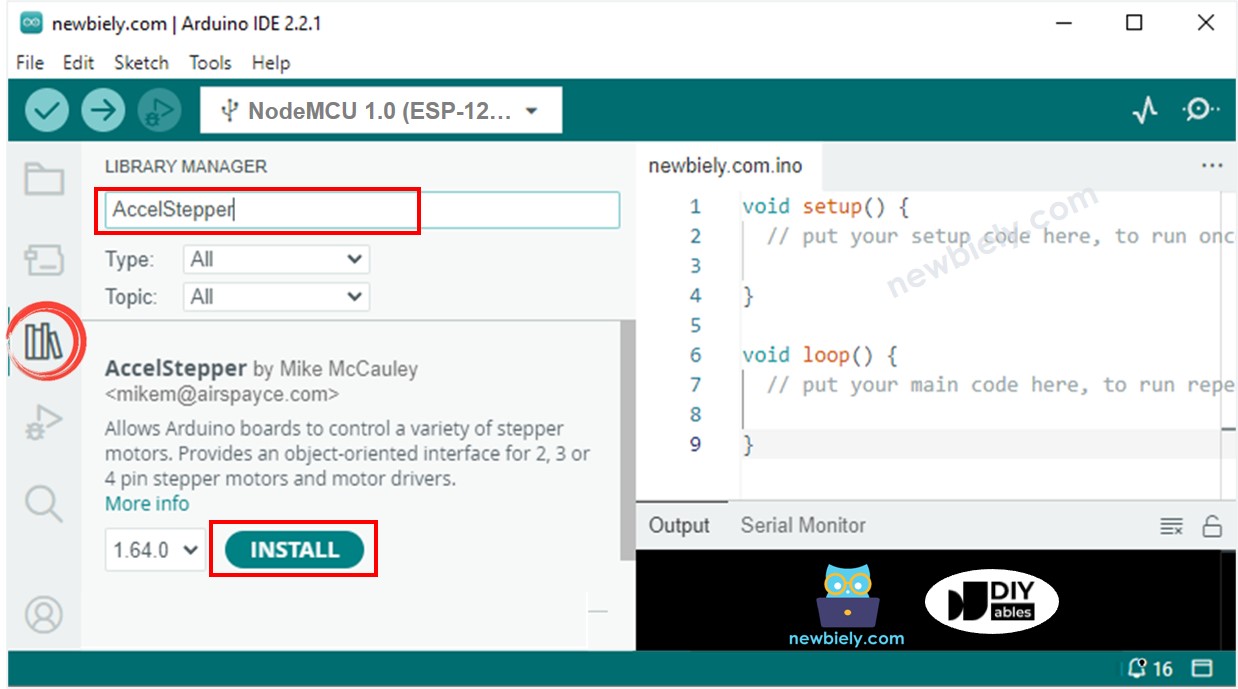
- Copy the code above and open it in Arduino IDE
- Click the Upload button in Arduino IDE to send the code to ESP8266
- You will observe the motor moving back and forth
When you use the motor in full-step mode, its movement might not be very smooth, which is normal. To make the motion smoother, turn on microstepping by setting the M1, M2, and M3 pins.